EDIT:
GAME RELEASED!
https://nikoichu.itch.io/midnight-fisherman
Hey, I'm making a fishing game (it's a spooky one don't worry).
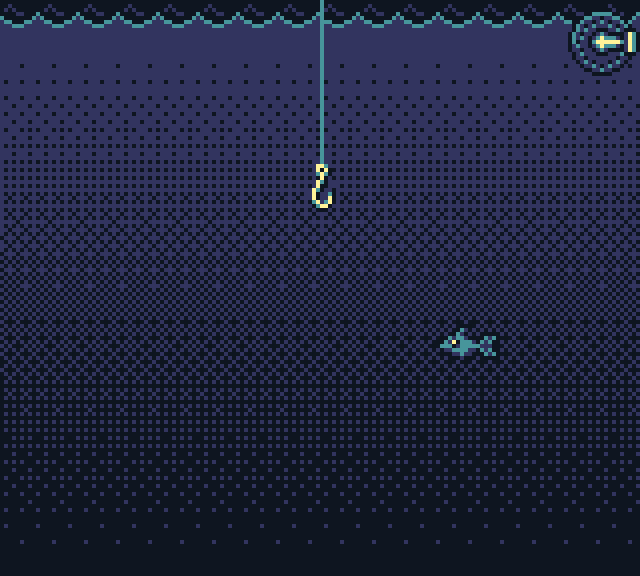
I was wondering what is allowed and what isn't in terms of asset creation.
-Are we allowed to use free images as a basis to trace our pixel art ontop of?
-Are we allowed to use free sounds to remix them into 8-bit sound effects?
-Is it required to make the sound effects and music sound 8-bit like they come from a gameboy, or can they be higher fidelity?
And a bonus one if anyone is familiar with Godot:
-Is there a way to enforce the 4-color palette via Shaders (or some other way?) and change it dynamically via code?