For completed projects you want the world to see, you can share code and/or screenshots here! Friendly reminder that Itch has a "code" format, which can prettify your posts and make things a bit more readable.
If your code was meant for a custom mode, include the CustomPreset.cps file for it, too. To install someone else's Custom preset, go to your game folder, create a new folder with the preset name (e.g, Compy6c), and drop the CustomPreset.cps file in (or make one, filling it with the appropriate contents - Windows users, make sure you can actually change the file extension on it!), then load it up in-game.
To get started, I'd like to share something straight off.
TCPaint
My crowning achievement so far in Senbir is a paint program written for Extended mode, which I'm currently referring to by the moniker "TCPaint". You NEED to be using the latest version, 0.43a.2 (I'll have a version text up on the main menu one day!), as there were some pretty crippling bugs (mainly in the monitor GetData function) I needed to fix in order for TCPaint to function.
//==PAINT== //WASD to navigate. Spacebar toggles if you're painting or not. Use E and Q to shift forwards and backwards through the list of colors, respectively. //This version was made for EXTENDED MODE, with its 16x8 resolution, and 4-color capability. //=REGISTER USAGE= //REGISTER 00: BRUSH DOWN? //REGISTER 01->2: KEY PRESS THIS TICK //REGISTER 03: USED FOR IFJMPS //REGISTER 04: BRUSH X //REGISTER 05: BRUSH Y //REGISTER 06: BACKGROUND COLOR REGISTER (USED IF BRUSH IS NOT DOWN) //REGISTER 07: BRUSH COLOR REGISTER (USED IF BRUSH IS DOWN) //REGISTER 08: TEMPORARY MODIFICATION REGISTER //REGISTER 12: INT 16 //REGISTER 13: INT 8 //REGISTER 14: INT 4 //REGISTER 15: INT 1 SET 15 3 00000001 //Set register 15 to the integer 01. Addr0. SET 14 3 00000100 //Set register 14 to the integer 04. Addr1. SET 13 3 00001000 //Set register 13 to the integer 08. Addr1. Yes, I know, there's a duplicate. SET 12 3 00010000 //Set register 12 to the integer 16. Addr2. SET 6 0 00000000 //Set register 6 to have the appropriate color. Addr3. SET 7 0 01000000 //Set register 7 to have the appropriate color - white. Addr4. GETDATA 2 3 0 //Get the last keyboard input. Addr5. PMOV 1 2 0 31 0 0 //Move the last keyboard input into register 2. Addr6. IFJMP 0 15 1 //If a key was pressed, jump to the input subroutine. Addr7. JMP 2 3 //Otherwise, do nothing. Addr8. PMOV 9 2 0 31 0 0 //Put the "brush down" variable in so IFJMP'll accept it. Addr9. IFJMP 0 2 0 //Jump to the background restore if the brush isn't down. Addr10. JMP 0 2 //Otherwise, jump past it. Addr11. SETDATA 0 3 6 //Wipe the old one. Addr12. PMOV 4 7 28 31 6 1 //Splice the last 4 bits of the X register (the actual x position) into the rendering register, offset +6 (<28>XXXX -> CCXXXX<26>). Addr13. PMOV 5 7 29 31 9 1 //Splice the last 3 bits of the Y register (the actual y position) into the rendering register, offset +9 (<29>YYY -> CCXXXXYYY<23>). Addr14. PMOV 7 8 0 31 30 1 //Addr15. GETDATA 0 3 8 //Get the monitor data. Addr16. MATH 1 6 5 //Paste the color in. Addr17. JMP 0 1 //Addr18. JMP 0 1 //Addr19. SETDATA 0 3 7 //Render the brush. Addr20. JMP 2 16 //Jump back to the start of the loop. Addr21. SET 0 0 01011101 //START OF INPUT SUBROUTINE. Set Register 0 to the key ID for W. Addr22. SET 0 1 11000000 //Set Register 0 to the key ID for W. Addr23. GETDATA 2 3 0 //Check the W. Addr24. MATH 1 2 5 //Move the result of GetData in. Addr25. IFJMP 0 34 1 //If W IS pressed, jump to the appropriate memory position. Addr26. SET 0 0 01011000 //Set Register 0 to the key ID for A. Addr27. SET 0 1 01000000 //Set Register 0 to the key ID for A. Addr28. GETDATA 2 3 0 //Check the A. Addr29. MATH 1 2 5 //Move the result of GetData in. Addr30. IFJMP 0 32 1 //If A IS pressed, jump to the appropriate memory position. Addr31. SET 0 0 01011100 //Set Register 0 to the key ID for S. Addr32. SET 0 1 11000000 //Set Register 0 to the key ID for S. Addr33. GETDATA 2 3 0 //Check the S. Addr34. MATH 1 2 5 //Move the result of GetData in. Addr35. IFJMP 0 30 1 //If S IS pressed, jump to the appropriate memory position. Addr36. SET 0 0 01011001 //Set Register 0 to the key ID for D. Addr37. SET 0 1 00000000 //Set Register 0 to the key ID for D. Addr38. GETDATA 2 3 0 //Check the D. Addr39. MATH 1 2 5 //Move the result of GetData in. Addr40. IFJMP 0 28 1 //If D IS pressed, jump to the appropriate memory position. Addr41. SET 0 0 01001000 //Set Register 0 to the key ID for SPACEBAR. Addr42. SET 0 1 00000000 //Set Register 0 to the key ID for SPACEBAR. Addr43. GETDATA 2 3 0 //Check the SPACEBAR. Addr44. MATH 1 2 5 //Move the result of GetData in. Addr45. IFJMP 0 26 1 //If SPACEBAR IS pressed, jump to the appropriate memory position. Addr46. SET 0 0 01011100 //Set Register 0 to the key ID for Q. Addr47. SET 0 1 01000000 //Set Register 0 to the key ID for Q. Addr48. GETDATA 2 3 0 //Check the Q. Addr49. MATH 1 2 5 //Move the result of GetData in. Addr50. IFJMP 0 28 1 //If Q IS pressed, jump to the appropriate memory position. Addr51. SET 0 0 01011001 //Set Register 0 to the key ID for E. Addr52. SET 0 1 01000000 //Set Register 0 to the key ID for E. Addr53. GETDATA 2 3 0 //Check the E. Addr54. MATH 1 2 5 //Move the result of GetData in. Addr55. IFJMP 0 33 1 //If E IS pressed, jump to the appropriate memory position. Addr56. SET 0 0 00000000 //Unset 0. Addr57. SET 0 1 00000000 //Unset 0. Addr58. JMP 2 50 //Jump back to the render loop. Addr59. MATH 15 5 1 //W WAS PRESSED. Increment Y down by one. Addr60. MATH 13 5 4 //Modulo Y just in case. Addr61. JMP 2 5 //Jump back to the render loop. Addr62. MATH 15 4 1 //A WAS PRESSED. Increment X down by one. Addr63. MATH 12 4 4 //Modulo Y just in case. Addr64. JMP 2 3 //Jump back to the render loop. Addr65. MATH 15 5 0 //S WAS PRESSED. Increment Y up by one. Addr66. MATH 13 5 4 //Modulo Y just in case. Addr67. JMP 2 3 //Jump back to the render loop. Addr68. MATH 15 4 0 //D WAS PRESSED. Increment X up by one. Addr69. MATH 12 4 4 //Modulo Y just in case. Addr70. JMP 2 3 //Jump back to the render loop. Addr71. MATH 9 2 5 //Move the contents of Address 9 (the brush toggle) into 2 for IFJMP use. Addr72. IFJMP 0 2 0 //Is the brush up? Addr73. JMP 0 3 //Nope. Addr74. SET 9 3 00000001 //Turn the brush on. Addr75. JMP 0 2 //Skip past turning it off. Addr76. SET 9 3 00000000 //Turn the brush off. Addr77. JMP 2 7 //Jump back to the render loop. Addr78. SET 8 0 00000000 //Addr79. SET 8 1 00000000 //Addr80. SET 8 2 00000000 //Addr81. SET 8 3 00000000 //Addr82. MATH 7 8 5 //Splice the color in. Addr83. PMOV 7 8 0 1 30 1 //THING. Addr84. MATH 15 8 1 //Q WAS PRESSED. Increment COL down by one. Addr85. MATH 14 8 4 //Modulo COL just in case. Addr86. PMOV 8 7 28 31 30 0 //Splice it back into the renderer. Addr87. JMP 2 10 //Jump back to the render loop. Addr88. SET 8 0 00000000 //Addr89. SET 8 1 00000000 //Addr90. SET 8 2 00000000 //Addr91. SET 8 3 00000000 //Addr92. MATH 7 8 5 //Splice the color in. Addr93. PMOV 7 8 0 1 30 1 //THING. Addr94. MATH 15 8 0 //E WAS PRESSED. Increment COL up by one. Addr95. MATH 14 8 4 //Modulo COL just in case. Addr96. PMOV 8 7 28 31 30 0 //Splice it back into the renderer. Addr97. JMP 2 10 //Jump back to the render loop. Addr98.
I'm really quite proud of it, and I think it's a good proof-of-concept to show that you legitimately can build anything in Senbir.
Use F to turn the computer on, then select the keyboard, then WASD to move your cursor, Q/E to cycle through the colors, and spacebar to toggle if your brush is "down" or not (effects whether or not it, well, paints, when you move the cursor) - it's simple, but it'll let you doodle some basic text messages or pixel art.
TCPaint (Extended Edition)
I've also been toying with a Custom preset for a much fancier computer (4kB of RAM, 32 kB drive, 6-bit 128x64 monitor with a proper RGB color palette, clockspeed 180hZ, the apparent maximum on my computer) and made an updated version of TCPaint for it.
CUSTOM PRESET (Compy6c/CustomPreset.cps)
1024 30000 6 7 0 0 0 0.3333333 0 0 0.6666667 0 0 1 0 0 0 0.3333333 0 0.3333333 0.3333333 0 0.6666667 0.3333333 0 1 0.3333333 0 0 0.6666667 0 0.2470588 0.6666667 0 0.6666667 0.6666667 0 1 0.6666667 0 0 1 0 0.3333333 1 0 0.6666667 1 0 1 1 0 0 0 0.3333333 0.3333333 0 0.3333333 0.6666667 0 0.3333333 1 0 0.3333333 0 0.3333333 0.3333333 0.3333333 0.3333333 0.3333333 0.6666667 0.3333333 0.3333333 1 0.3333333 0.3333333 0 0.6666667 0.3333333 0.3333333 0.6666667 0.3333333 0.6666667 0.6666667 0.3333333 1 0.6666667 0.3333333 0 1 0.3333333 0.3333333 1 0.3333333 0.6666667 1 0.3333333 1 1 0.3333333 0 0 0.6666667 0.3333333 0 0.6666667 0.6666667 0 0.6666667 1 0 0.6666667 0 0.3333333 0.6666667 0.3333333 0.3333333 0.6666667 0.6666667 0.3333333 0.6666667 1 0.3333333 0.6666667 0 0.6666667 0.6666667 0.3333333 0.6666667 0.6666667 0.6666667 0.6666667 0.6666667 1 0.6666667 0.6666667 0 1 0.6666667 0.3333333 1 0.6666667 0.6666667 1 0.6666667 1 1 0.6666667 0 0 1 0.3333333 0 1 0.6666667 0 1 1 0 1 0 0.3333333 1 0.3333333 0.3333333 1 0.6666667 0.3333333 1 1 0.3333333 1 0 0.6666667 1 0.3333333 0.6666667 1 0.6666667 0.6666667 1 1 0.6666667 1 0 1 1 0.3333333 1 1 0.6666667 1 1 1 1 1 False 8192
TCPAINT ITSELF (Paint.casm)
//==PAINT== //WASD to navigate. Spacebar toggles if you're painting or not. Use E and Q to shift forwards and backwards through the list of colors, respectively. //This version was made for the COMPY-6C, with its 128x64 resolution, and 64-color capability. //=REGISTER USAGE= //REGISTER 00: BRUSH DOWN? //REGISTER 01->2: KEY PRESS THIS TICK //REGISTER 03: USED FOR IFJMPS //REGISTER 04: BRUSH X //REGISTER 05: BRUSH Y //REGISTER 06: BACKGROUND COLOR REGISTER (USED IF BRUSH IS NOT DOWN) //REGISTER 07: BRUSH COLOR REGISTER (USED IF BRUSH IS DOWN) //REGISTER 08: TEMPORARY MODIFICATION REGISTER //REGISTER 12: INT 128 //REGISTER 13: INT 64 //REGISTER 14: INT 64 //REGISTER 15: INT 1 SET 15 3 00000001 //Set register 15 to the integer 01. Addr0. SET 14 3 01000000 //Set register 14 to the integer 64. Addr1. SET 13 3 01000000 //Set register 13 to the integer 64. Addr1. SET 12 3 10000000 //Set register 12 to the integer 128. Addr2. SET 6 0 00000000 //Set register 6 to have the appropriate color. Addr3. SET 7 0 11111100 //Set register 7 to have the appropriate color - white. Addr4. GETDATA 2 3 0 //Get the last keyboard input. Addr5. PMOV 1 2 0 31 0 0 //Move the last keyboard input into register 2. Addr6. IFJMP 0 15 1 //If a key was pressed, jump to the input subroutine. Addr7. JMP 2 3 //Otherwise, do nothing. Addr8. PMOV 9 2 0 31 0 0 //Put the "brush down" variable in so IFJMP'll accept it. Addr9. IFJMP 0 2 0 //Jump to the background restore if the brush isn't down. Addr10. JMP 0 2 //Otherwise, jump past it. Addr11. SETDATA 0 3 6 //Wipe the old one. Addr12. PMOV 4 7 25 31 13 1 //Splice the last 7 bits of the X register (the actual x position) into the rendering register, offset +6 (<28>XXXX -> CCXXXX<26>). Addr13. PMOV 5 7 26 31 19 1 //Splice the last 6 bits of the Y register (the actual y position) into the rendering register, offset +9 (<29>YYY -> CCXXXXYYY<23>). Addr14. PMOV 7 8 0 31 26 1 //Addr15. GETDATA 0 3 8 //Get the monitor data. Addr16. MATH 1 6 5 //Paste the color in. Addr17. JMP 0 1 //Addr18. JMP 0 1 //Addr19. SETDATA 0 3 7 //Render the brush. Addr20. JMP 2 16 //Jump back to the start of the loop. Addr21. SET 0 0 01011101 //START OF INPUT SUBROUTINE. Set Register 0 to the key ID for W. Addr22. SET 0 1 11000000 //Set Register 0 to the key ID for W. Addr23. GETDATA 2 3 0 //Check the W. Addr24. MATH 1 2 5 //Move the result of GetData in. Addr25. IFJMP 0 34 1 //If W IS pressed, jump to the appropriate memory position. Addr26. SET 0 0 01011000 //Set Register 0 to the key ID for A. Addr27. SET 0 1 01000000 //Set Register 0 to the key ID for A. Addr28. GETDATA 2 3 0 //Check the A. Addr29. MATH 1 2 5 //Move the result of GetData in. Addr30. IFJMP 0 32 1 //If A IS pressed, jump to the appropriate memory position. Addr31. SET 0 0 01011100 //Set Register 0 to the key ID for S. Addr32. SET 0 1 11000000 //Set Register 0 to the key ID for S. Addr33. GETDATA 2 3 0 //Check the S. Addr34. MATH 1 2 5 //Move the result of GetData in. Addr35. IFJMP 0 30 1 //If S IS pressed, jump to the appropriate memory position. Addr36. SET 0 0 01011001 //Set Register 0 to the key ID for D. Addr37. SET 0 1 00000000 //Set Register 0 to the key ID for D. Addr38. GETDATA 2 3 0 //Check the D. Addr39. MATH 1 2 5 //Move the result of GetData in. Addr40. IFJMP 0 28 1 //If D IS pressed, jump to the appropriate memory position. Addr41. SET 0 0 01001000 //Set Register 0 to the key ID for SPACEBAR. Addr42. SET 0 1 00000000 //Set Register 0 to the key ID for SPACEBAR. Addr43. GETDATA 2 3 0 //Check the SPACEBAR. Addr44. MATH 1 2 5 //Move the result of GetData in. Addr45. IFJMP 0 26 1 //If SPACEBAR IS pressed, jump to the appropriate memory position. Addr46. SET 0 0 01011100 //Set Register 0 to the key ID for Q. Addr47. SET 0 1 01000000 //Set Register 0 to the key ID for Q. Addr48. GETDATA 2 3 0 //Check the Q. Addr49. MATH 1 2 5 //Move the result of GetData in. Addr50. IFJMP 0 28 1 //If Q IS pressed, jump to the appropriate memory position. Addr51. SET 0 0 01011001 //Set Register 0 to the key ID for E. Addr52. SET 0 1 01000000 //Set Register 0 to the key ID for E. Addr53. GETDATA 2 3 0 //Check the E. Addr54. MATH 1 2 5 //Move the result of GetData in. Addr55. IFJMP 0 33 1 //If E IS pressed, jump to the appropriate memory position. Addr56. SET 0 0 00000000 //Unset 0. Addr57. SET 0 1 00000000 //Unset 0. Addr58. JMP 2 50 //Jump back to the render loop. Addr59. MATH 15 5 1 //W WAS PRESSED. Increment Y down by one. Addr60. MATH 13 5 4 //Modulo Y just in case. Addr61. JMP 2 5 //Jump back to the render loop. Addr62. MATH 15 4 1 //A WAS PRESSED. Increment X down by one. Addr63. MATH 12 4 4 //Modulo Y just in case. Addr64. JMP 2 3 //Jump back to the render loop. Addr65. MATH 15 5 0 //S WAS PRESSED. Increment Y up by one. Addr66. MATH 13 5 4 //Modulo Y just in case. Addr67. JMP 2 3 //Jump back to the render loop. Addr68. MATH 15 4 0 //D WAS PRESSED. Increment X up by one. Addr69. MATH 12 4 4 //Modulo Y just in case. Addr70. JMP 2 3 //Jump back to the render loop. Addr71. MATH 9 2 5 //Move the contents of Address 9 (the brush toggle) into 2 for IFJMP use. Addr72. IFJMP 0 2 0 //Is the brush up? Addr73. JMP 0 3 //Nope. Addr74. SET 9 3 00000001 //Turn the brush on. Addr75. JMP 0 2 //Skip past turning it off. Addr76. SET 9 3 00000000 //Turn the brush off. Addr77. JMP 2 7 //Jump back to the render loop. Addr78. SET 8 0 00000000 //Addr79. SET 8 1 00000000 //Addr80. SET 8 2 00000000 //Addr81. SET 8 3 00000000 //Addr82. MATH 7 8 5 //Splice the color in. Addr83. PMOV 7 8 0 5 26 1 //THING. Addr84. MATH 15 8 1 //Q WAS PRESSED. Increment COL down by one. Addr85. MATH 14 8 4 //Modulo COL just in case. Addr86. PMOV 8 7 24 31 26 0 //Splice it back into the renderer. Addr87. JMP 2 10 //Jump back to the render loop. Addr88. SET 8 0 00000000 //Addr89. SET 8 1 00000000 //Addr90. SET 8 2 00000000 //Addr91. SET 8 3 00000000 //Addr92. MATH 7 8 5 //Splice the color in. Addr93. PMOV 7 8 0 5 26 1 //THING. Addr94. MATH 15 8 0 //E WAS PRESSED. Increment COL up by one. Addr95. MATH 14 8 4 //Modulo COL just in case. Addr96. PMOV 8 7 24 31 26 0 //Splice it back into the renderer. Addr97. JMP 2 10 //Jump back to the render loop. Addr88.
NOTES
The color palette is ordered from least blue to most blue, then least green to most green, then least red to most red. Color slot 1 is a dim red, while 3 is bright. Color slot 5 is a dim yellow, combining both dim red and dim green. Etc. Every 16 colors, the blue level increases.
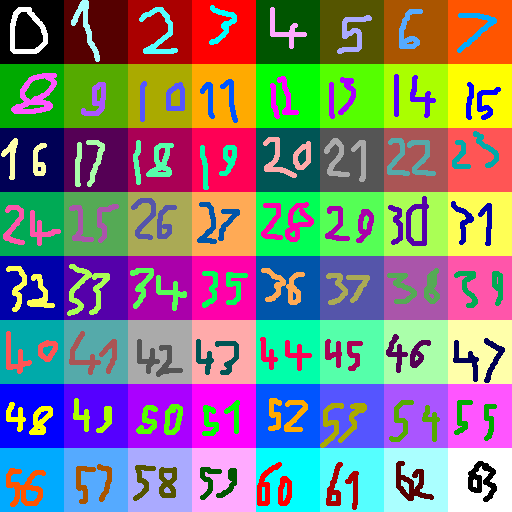